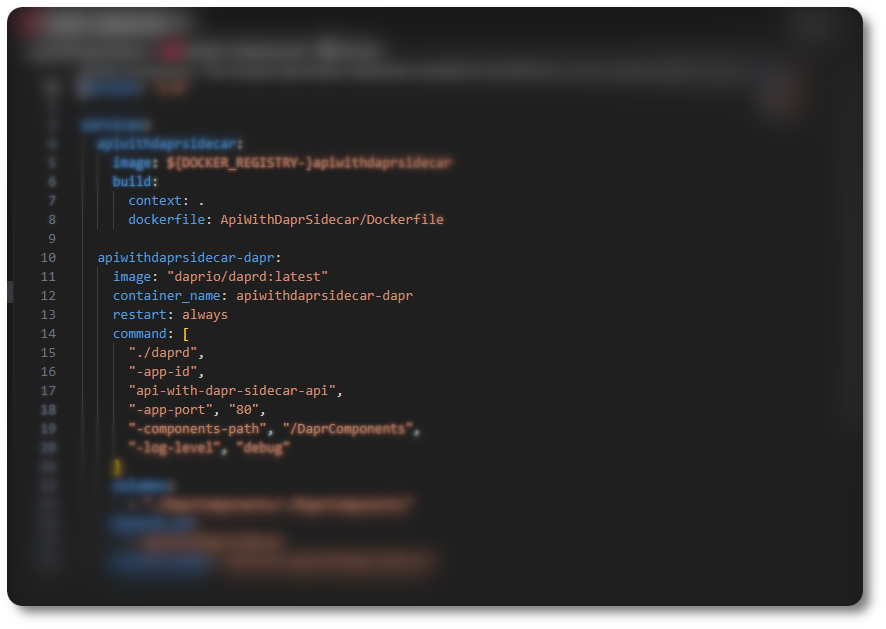
Dapr Sidecar with docker-compose
Dapr Sidecar with docker-compose
The Problem
When following the Dapr Getting started guide and quickstarts, the context is from Dapr point of view. The apps are “Dapr apps” running via the dapr CLI. I want the API to be the central point of focus so that developers on my team open the solution and simply run the app, with Dapr acting as a true sidecar.
Background
TL;DR: Use docker-compose to run Dapr as a sidecar
When I started evaluating Azure Container Apps and Dapr as possible suite of tools for developing a new system, I began by following the Dapr Getting started guide. Following the guide is helpful to quickly get up and running to evaluate the tools - will this do what I would like it to do, and does it provide the necessary functionality in an easily consumable way for other developers on my team? In my case - the answer was yes.
Next, I created a proof-of-concept application in Azure Container Apps. I built out two APIs which utilized the Dapr pubsub component and had these APIs communicate through pubsub. I found this really great Bit of Technology series of blog posts extremely helpful. Head over that way if you are interested in configuring Container Apps, observability, and repeatable deployment processes!
The challenge at this point was to come up with a pleasant developer experience. The goal is for a developer to pull down any given API and work on that API independently of other APIs running within our toolset (Visual Studio / VS Code in our case). The API should just work without using the Dapr CLI commands.
As long as the API subscribes to the relevant topics, handles the messages according to the business logic, publishes the appropriate messages, and has a comprehensive suite of unit tests, the work for that API should be done. Integration validation can happen once the application is deployed to Azure. With that said, sometimes it can be convenient to run a couple apps at the same time to test specific use cases, so how can I setup the API to run with Dapr locally while providing a good developer experience?
Application Setup with docker-compose
Check out the repo which contains all the code referenced in this post!
At this point, I’m going to walk through an example to setup an application with Dapr as a sidecar. I’ll be using Visual Studio to create a .NET Web API project, but the concepts will still apply and will work if your application is launched with a docker-compose file.
- In Visual Studio, create a new project
- Select the “ASP.NET Core Web API” template
- Give the project name and set other configuration values as needed
- Ensure the “Enable Docker” checkbox is selected
- Click “Create”
- Open the solution
- Right click on the API project and select “Add”, then select “Container Orchestrator Support”
- The dropdown should say “Docker Compose”, click “OK”
- Select the target OS and click “OK”
- Right click on “docker-compose” in the Solution Explorer and select “Set as Startup Project”
- Add the following service to docker-compose.yml, under the default service*:
apiwithdaprsidecar-dapr: image: "daprio/daprd:latest" container_name: apiwithdaprsidecar-dapr restart: always command: [ "./daprd", "-app-id", "api-with-dapr-sidecar-api", "-app-port", "80", "-components-path", "/DaprComponents", "-log-level", "debug" ] volumes: - "./DaprComponents/:/DaprComponents" depends_on: - apiwithdaprsidecar network_mode: "service:apiwithdaprsidecar"
- Now in Visual Studio, click the green play button (it should say “Docker Compose” if you did step 10 above). You should
have two containers running that look something like this:
Note*: Regarding the components-path and volumes in the docker-compose file, I prefer to store local development specific Dapr components for each API in a DaprComponents folder.
Wrapping It Up
At this point, the API is ready for any developer to pick up, start writing code, and running locally. The development experience is on the app itself, with little to no thought about Dapr running along in the background. The docker-compose file is just for local development convenience. For deployment to Azure Container Apps, the API will be built as a Docker image, pushed to a container registry, and deployed to a Container Apps Environment as a new container app or a revision to an existing container app.
Check out the followup article on how to authenticate and authorize Dapr requests in a .NET Web API project with the Dapr .NET SDK!